Demographics for Canadian Websites
neighbourhood.io API lets you embed Canadian neighbourhood information by Geolocation in your web pages.
Our WebAPI includes age distribution, marital status of residents, structural types of housing, owned/rented properties, average household income and much more.
neighbourhood.io contains data compiled from several Statistics Canada sources
Population
Age, sex, race, origin, labor force, educational attainment, marital status
Income
Household income, per capita income, age by income, disposable income, net worth
Households
Total households, total family households, average household size
Housing
Housing—Such as home value, owner-occupied units, renter-occupied units, vacant units
Easy to Use Restful API
We have designed the neighbourhood.io API in a very RESTful way. All actions and relationships are clearly documented and users can often get up and running with the API in less than ten minutes.
Complete Code Samples in JavaScript, C#, PHP Python, Ruby, Curl, Java, Objective C.
1
2
3
4
5
|
@ECHO OFF REM for Basic Authorization use: --user {username}:{password} REM Specify values for path parameters (shown as {...}), your subscription key and values for query parameters |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
<!DOCTYPE html> < html > < head > < title >JSSample</ title > </ head > < body > < script type = "text/javascript" > $(function() { var params = { // Specify your subscription key 'subscription-key': '', }; $.ajax({ url: 'https://neighbourhood.azure-api.net/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}?' + $.param(params), type: 'GET', }) .done(function(data) { alert("success"); }) .fail(function() { alert("error"); }); }); </ script > </ body > </ html > |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
using System; using System.Net.Http.Headers; using System.Text; using System.Net.Http; using System.Web; namespace CSHttpClientSample { static class Program { static void Main() { MakeRequest(); Console.WriteLine( "Hit ENTER to exit..." ); Console.ReadLine(); } static async void MakeRequest() { var client = new HttpClient(); var queryString = HttpUtility.ParseQueryString( string .Empty); /* Basic Authorization Sample client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("basic", Convert.ToBase64String(Encoding.UTF8.GetBytes("{username}:{password}"))); */ // Specify your subscription key queryString[ "subscription-key" ] = "" ; // Specify values for path parameters (shown as {...}) var uri = "https://neighbourhood.azure-api.net/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}?" + queryString; var response = await client.GetAsync(uri); if (response.Content != null ) { var responseString = await response.Content.ReadAsStringAsync(); Console.WriteLine(responseString); } } } } |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
<?php // This sample uses the HTTP_Request2 package. (for more information: http://pear.php.net/package/HTTP_Request2) require_once 'HTTP/Request2.php' ; $headers = array ( ); $query_params = array ( // Specify your subscription key 'subscription-key' => '' , ); $request = new Http_Request2( 'https://neighbourhood.azure-api.net/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}' ); $request ->setMethod(HTTP_Request2::METHOD_GET); // Basic Authorization Sample // $request-setAuth('{username}', '{password}'); $request ->setHeader( $headers ); $url = $request ->getUrl(); $url ->setQueryVariables( $query_params ); try { $response = $request ->send(); echo $response ->getBody(); } catch (HttpException $ex ) { echo $ex ; } ?> |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
########### Python 2.7 ############# import httplib, urllib, base64 headers = { # Basic Authorization Sample # 'Authorization': 'Basic %s' % base64.encodestring('{username}:{password}'), } params = urllib.urlencode({ # Specify your subscription key 'subscription-key' : '', }) try : conn = httplib.HTTPSConnection( 'neighbourhood.azure-api.net' ) conn.request( "GET" , "/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}?%s" % params, "", headers) response = conn.getresponse() data = response.read() print (data) conn.close() except Exception as e: print ( "[Errno {0}] {1}" . format (e.errno, e.strerror)) #################################### ########### Python 3.2 ############# import http.client, urllib.request, urllib.parse, urllib.error, base64 headers = { # Basic Authorization Sample # 'Authorization': 'Basic %s' % base64.encodestring('{username}:{password}'), } params = urllib.parse.urlencode({ # Specify your subscription key 'subscription-key' : '', }) try : conn = http.client.HTTPSConnection( 'neighbourhood.azure-api.net' ) conn.request( "GET" , "/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}?%s" % params, "", headers) response = conn.getresponse() data = response.read() print (data) conn.close() except Exception as e: print ( "[Errno {0}] {1}" . format (e.errno, e.strerror)) #################################### |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
require 'net/http' uri = URI ( 'https://neighbourhood.azure-api.net/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}' ) uri.query = URI .encode_www_form({ # Specify your subscription key 'subscription-key' => '' , }) request = Net:: HTTP ::Get. new (uri.request_uri) # Basic Authorization Sample # request.basic_auth 'username', 'password' response = Net:: HTTP .start(uri.host, uri.port, :use_ssl => uri.scheme == 'https' ) do |http| http.request(request) end puts response.body |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
// This sample uses the Apache HTTP client from HTTP Components (http://hc.apache.org/httpcomponents-client-ga/) import java.net.URI; import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.utils.URIBuilder; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; public class JavaSample { public static void main(String[] args) { HttpClient httpclient = HttpClients.createDefault(); try { URIBuilder builder = new URIBuilder( "https://neighbourhood.azure-api.net/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}" ); // Specify your subscription key builder.setParameter( "subscription-key" , "" ); URI uri = builder.build(); HttpGet request = new HttpGet(uri); HttpResponse response = httpclient.execute(request); HttpEntity entity = response.getEntity(); if (entity != null ) { System.out.println(EntityUtils.toString(entity)); } } catch (Exception e) { System.out.println(e.getMessage()); } } } |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
|
#import <Foundation/Foundation.h> int main( int argc, const char * argv[]) { NSAutoreleasePool * pool = [[ NSAutoreleasePool alloc] init]; NSString * path = @ "https://neighbourhood.azure-api.net/neighbourhood/v1?Latitude={Latitude}&Longitude={Longitude}" ; NSArray * array = @[ // Specify your subscription key @ "subscription-key=" , @ "entities=true" , ]; NSString * string = [array componentsJoinedByString:@ "&" ]; path = [path stringByAppendingFormat:@ "?%@" , string]; NSLog (@ "%@" , path); NSMutableURLRequest * _request = [ NSMutableURLRequest requestWithURL:[ NSURL URLWithString:path]]; [_request setHTTPMethod:@ "GET" ]; NSURLResponse *response = nil ; NSError *error = nil ; NSData * _connectionData = [ NSURLConnection sendSynchronousRequest:_request returningResponse:&response error:&error]; if ( nil != error) { NSLog (@ "Error: %@" , error); } else { NSError * error = nil ; NSMutableDictionary * json = nil ; NSString * dataString = [[ NSString alloc] initWithData:_connectionData encoding: NSUTF8StringEncoding ]; NSLog (@ "%@" , dataString); if ( nil != _connectionData) { json = [ NSJSONSerialization JSONObjectWithData:_connectionData options: NSJSONReadingMutableContainers error:&error]; } if (error || !json) { NSLog (@ "Could not parse loaded json with error:%@" , error); } NSLog (@ "%@" , json); _connectionData = nil ; } [pool drain]; return 0; } |
Chartjs Sample
Chartjs Sample using Neighbourhood API.
Martial Status
Age Distribution
Household income
Google Chart Sample
Google Chart Sample using Neighbourhood API.
Mother Tounge
Gender
Education
amChart Sample
amChart using Neighbourhood API.
Construction
Employment Type
Income
Starter
- Standard Support
- 1000 API calls / day
- 1 Province
- Restful API
- Domain Restriction
- Developer Portal
Pro
Best Value- Silver Support
- 25,000 API calls / day
- All Provinces
- Restful API
- IP Restriction
- Developer Portal Access
Enterprise
- Gold Support
- 100,000 API calls / day
- All Provinces
- Restful API
- No Restrictions
- Developer Portal Access
Our Customers
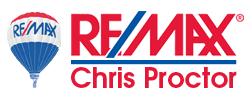
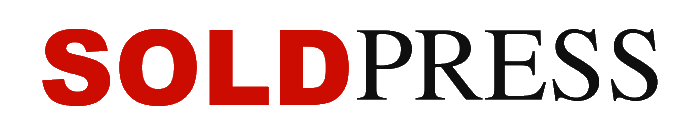
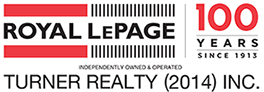
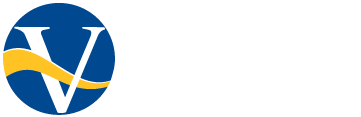
Frequently Asked Questions
What kind of support do I get with each plan?
We offer a tiered support level with each plan that is tailored to your specific needs
Support Level | What’s included |
---|---|
Standard | Email (24 Hours) |
Silver | Email (4 Hours) |
Gold | Email and phone support |
What is a Dissemination area
A dissemination area (DA) is a small, relatively stable geographic unit composed of one or more adjacent dissemination blocks. It is the smallest standard geographic area for which all census data are disseminated. DAs cover all the territory of Canada. Small area composed of one or more neighbouring dissemination blocks, with a population of 400 to 700 persons. All of Canada is divided into dissemination areas.
What are the data segments currently available?
Age, Gender,Mother Tongue ,Marital Status,Education,Employment,Income,Religion
What is an "API call"?
Each HTTP GET request that you successfully make to the neighbourhood.io servers is counted as an API call. Your calls are uniquely identified by means of the API key that is assigned to you upon sign-up.
How do I make payments?
You can use your credit card to make your payment. All payments are handled through Stripe and are completely secure
How can I access statements and invoices?
All invoices are sent by email.
Don't Be Shy
If we didn’t answer all of your questions, feel free to drop us a line anytime.